Summer ’25 is almost here to deliver powerful enhancements across Flow and Orchestration that help admins, developers, and business users build faster, smarter, and more scalable automations. Whether you’re deep into Flow Builder daily or just dipping your toes into automation, there’s something in this release for you. Let’s explore what’s new, along with use […]
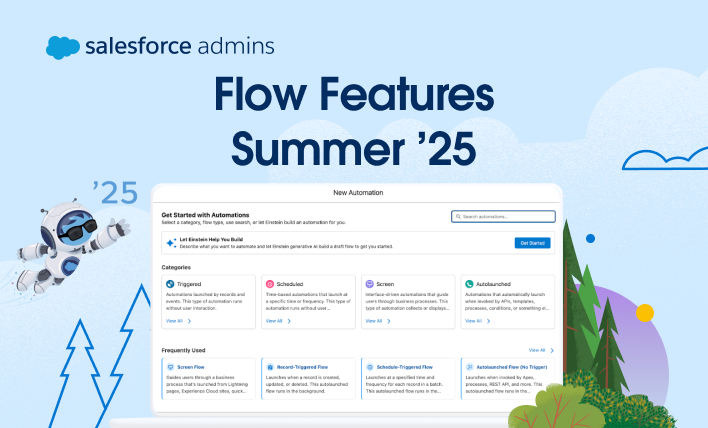